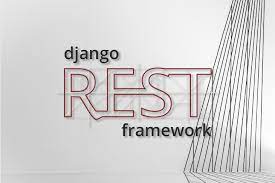
Django REST Framework (DRF) is a powerful and flexible toolkit for building Web APIs. It is an integral part of many Django projects, enabling developers to create robust and scalable RESTful APIs. However, working with DRF can sometimes present challenges, especially when encountering unexpected issues. Whether you are a part of a Django development company or an individual developer, having strong troubleshooting skills is essential. This guide will walk you through common issues and their solutions, helping you to efficiently resolve problems and optimize your DRF projects. Leveraging Django development services can also provide additional expertise to streamline your process.
Common Setup Issues
Incorrect Installation
One of the first hurdles developers face is ensuring DRF is installed correctly. Issues can arise from version conflicts or incomplete installations. To avoid this, always follow the official installation guide and verify the installation by checking that all dependencies are correctly installed.
Configuration Errors
Proper configuration is crucial for DRF to function correctly. Configuration errors can stem from incorrect settings in the settings.py file. Ensure that the necessary DRF components are included and that middleware settings are appropriately configured.
Dependency Conflicts
DRF relies on several dependencies that must be compatible with your Django version. Conflicts can cause significant issues. Using a virtual environment and a requirements file can help manage dependencies effectively. This ensures all required packages are installed correctly.
Authentication and Authorization Problems
Token-Based Authentication Issues
Authentication is a critical aspect of any API. Token-based authentication is widely used in DRF, but it can present issues if not configured properly. Ensure that your authentication classes are correctly set in your configuration.
Permissions and Access Control Problems
Permissions define who can access your API endpoints. Misconfigured permissions can lead to unauthorized access or restricted functionality. Use DRF's built-in permissions and ensure they are correctly implemented in your views.
Serialization Errors
Incorrect Serializer Usage
Serializers in DRF handle the conversion between complex data types and JSON. Using serializers incorrectly can lead to validation errors and improper data handling. Ensure your serializers are defined correctly and include all necessary fields.
Validation Issues
Validation errors are common when working with serializers. Custom validators can help ensure data integrity. Properly handle and validate data to prevent errors.
Handling Nested Serializers
Nested serializers can complicate data serialization and deserialization. Properly handle nested relationships to avoid data integrity issues. Use appropriate methods to manage nested data.
View and URL Configuration Troubles
ViewSet and Router Mismatches
Using ViewSets and Routers simplifies URL routing, but mismatches can lead to broken endpoints. Ensure that your ViewSets are correctly registered with routers and that URL patterns match the views they are intended to trigger.
URL Patterns Not Matching
URL patterns must match the views they are intended to trigger. Double-check your URLs to ensure correct mapping of paths to views.
Incorrect HTTP Methods
DRF views must handle the correct HTTP methods (GET, POST, PUT, DELETE). Ensure your views explicitly define allowed methods and handle them appropriately.
Database and ORM Issues
Query Optimization Problems
Inefficient queries can slow down your API. Use Django's ORM effectively to optimize queries. Prefetch related objects and use annotations for calculations to improve performance.
Handling Related Models
Managing related models in DRF requires careful handling to maintain data integrity. Use serializers to manage relationships properly and ensure data is handled correctly.
Managing Database Migrations
Database migrations can be tricky, especially with large projects. Ensure you run migrations in the correct order and resolve any conflicts promptly.
Performance Bottlenecks
Identifying Slow Endpoints
Identifying slow endpoints is the first step in performance optimization. Use Django's built-in tools and third-party libraries to analyze performance and identify bottlenecks.
Optimizing QuerySets
Optimizing querysets is crucial for performance. Use methods to reduce database hits and improve response times.
Caching Strategies
Implement caching strategies to reduce database load and improve response times. Use caching frameworks or third-party tools to enhance performance.
Testing and Debugging
Writing Effective Tests
Writing effective tests ensures your API works as expected. Use Django's test framework to create unit tests for your views, serializers, and models.
Using Debugging Tools
Debugging tools help identify and fix issues quickly. Use these tools to step through code and inspect variables to find the root cause of problems.
Analyzing Error Logs
Error logs provide valuable insights into issues. Regularly check your logs and use them to troubleshoot problems and understand what went wrong.
Deployment Challenges
Configuration for Production
Deploying a DRF project to production requires careful configuration. Ensure your settings are optimized for security and performance to handle production traffic.
Dealing with Environment-Specific Issues
Environment-specific issues can arise during deployment. Use environment variables and configuration files to manage different settings for development, staging, and production environments.
Monitoring and Logging
Monitoring and logging are essential for maintaining a healthy application. Use tools to monitor performance and log errors to keep track of the application's health.
Conclusion
Troubleshooting Django REST Framework issues requires a systematic approach and a deep understanding of both Django and DRF. Whether you are part of a Django development company or an individual developer, mastering these troubleshooting techniques will enhance your ability to build and maintain robust APIs. By addressing common setup issues, authentication problems, serialization errors, and performance bottlenecks, you can ensure your DRF projects run smoothly and efficiently. Additionally, utilizing Django development services can provide expert guidance and support, further streamlining your development process.