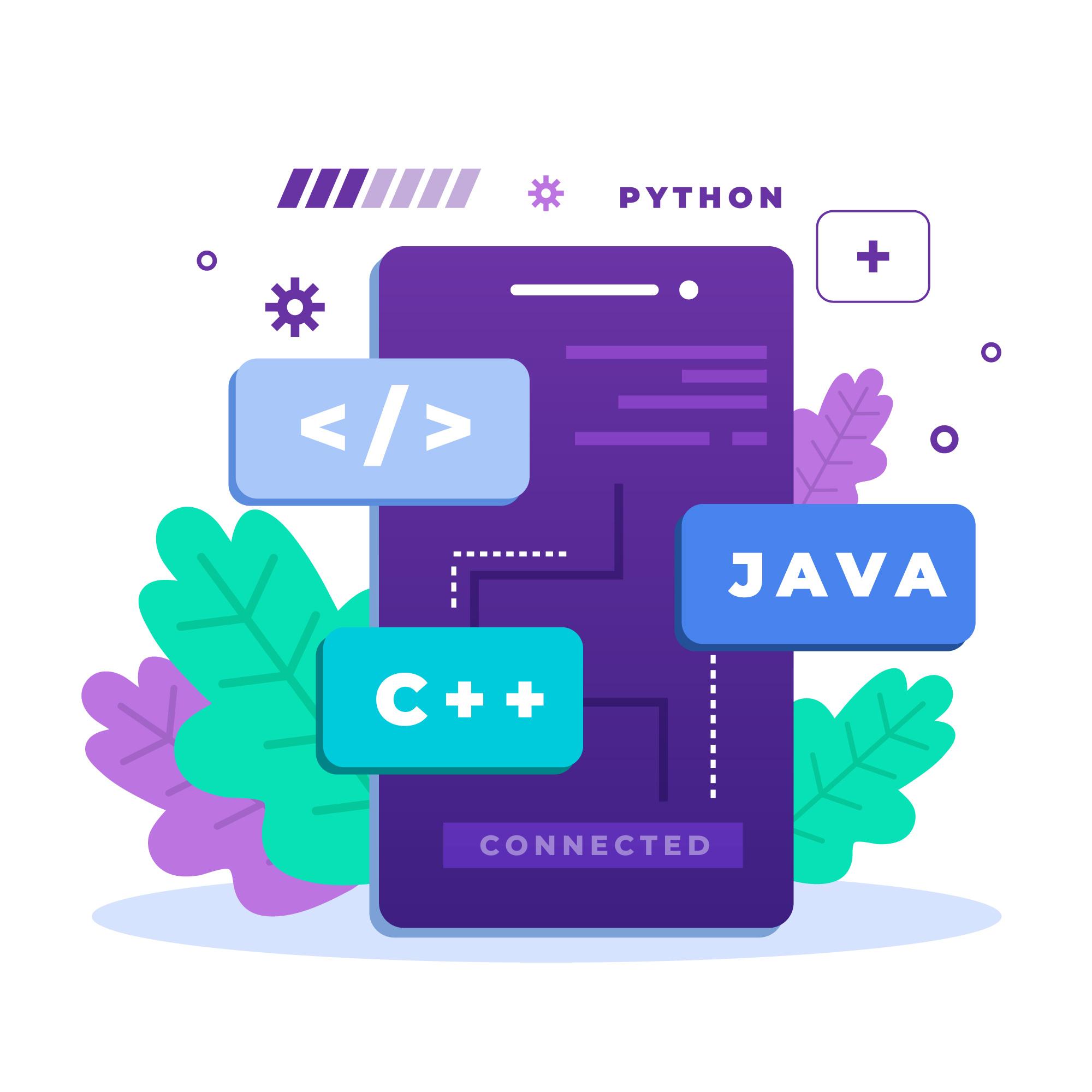
Dealing with different data types in C++ is quite general. But have you ever tried to add an integer and a float in C++? You would have definitely faced an error due to a type mismatch.
You would wonder, "Why can I not just add them together?" or "What is going on with my data?
This is where type conversion in C++ steps in—like making sure all the pieces of your puzzle fit together.
It could be anything from adding an `int` to a `double` or dealing with complex objects and pointers; type conversion will save you and ensure things work correctly. However, it can lead to subtle bugs, data loss, or unexpected behaviour if it is used without a proper understanding.
In C++ type conversion refers to the method by which changes can be performed to the data and turn from one type to the other. It ensures that each operation mixing different types is correctly executed.
Overview of Type Conversion in C++
In C++, an explicit conversion is referred to as type conversion through which a variable is converted to another data type. This will become essential when we want to use or process different kinds of data together.
Imagine that you have an int and a float, and we want to add them. We cannot simply throw them together and pray for the best. C++ needs to be told how to handle these different types.
There are two main types of conversions:
- Implicit Type Conversion (Automatic)
- Explicit Type Conversion (Manual or Type Casting)
Knowing both implicit and explicit type casting in C++ tutorial will not only save you from errors but also help in writing cleaner and more efficient code.
Both of these have their own purposes, and using them at the right time can save us from a lot of headaches.
Implicit Type Conversion in C++
Implicit type conversion is where the C++ compiler does the heavy lifting for us. We don’t have to worry about converting types manually.
The compiler sees that we’re mixing types and automatically promotes the smaller type to match the larger one. This is often called “type promotion.”
For instance, when adding an int to a double, the int is automatically converted to a double to ensure the operation goes smoothly.
Example 1: Conversion from int to double in an Expression
#include using namespace std;
int main() { int wholeNumber = 10; double decimalNumber = 5.5;
double result = wholeNumber + decimalNumber; cout << "Result: " << result << endl; return 0; } |
Output:
Result: 15.5 |
In this example, C++ automatically converts the int whole number to a double before performing the addition.
Example 2: Conversion from char to int using Implicit Type Promotion
#include using namespace std; int main() { char letter = 'A'; // ASCII value of 'A' is 65 int sum = letter + 5; cout << "Sum: " << sum << endl;
return 0; } |
Output:
Sum: 70 |
Here, the char is implicitly converted to its ASCII int value (65), allowing us to perform arithmetic operations without errors.
Explicit Type Conversion in C++ (Type Casting)
We often need more control over how our data is converted in C++. Sometimes, the compiler’s automatic conversion just won’t cut it.
That’s where explicit type conversion comes into play. This is all about telling the compiler exactly how we want our data to be treated.
When we use explicit conversion, we’re taking matters into our own hands, ensuring that the data types are exactly what we need.
Static Cast in C++ and When to Use It
Static_cast is the go-to when we need to convert between related types at compile time. It’s useful for straightforward conversions where we’re sure of what we’re doing.
For example, converting a float to an int or casting between pointers of related classes.
Example: Using Static Cast to Convert a float to int
#include using namespace std;
int main() { float price = 19.99; int roundedPrice = static_cast
cout << "Rounded Price: " << roundedPrice << endl;
return 0; } |
Output:
Rounded Price: 19 |
In this case, static_cast is used to convert the float price to an int. This is great when dealing with a whole number, and we do not care about the fractional part.
Dynamic Cast in C++ for Safe Downcasting
Dynamic_cast is essential when working with inheritance and we need to safely cast from a base class to a derived class.
It’s a runtime check, so if the cast isn’t valid, it returns nullptr or throws an error, depending on what we’re doing.
This makes it safer than static_cast when dealing with polymorphism.
Example: Utilizing Dynamic Cast for Base to Derived Class Conversion
#include using namespace std; class Base { public: virtual void show() { cout << "Base class" << endl; } };
class Derived : public Base { public: void show() override { cout << "Derived class" << endl; } };
int main() { Base* basePtr = new Derived(); Derived* derivedPtr = dynamic_cast
if (derivedPtr) { derivedPtr->show(); } else { cout << "Invalid cast" << endl; }
return 0; } |
Output:
Derived class |
Here, dynamic_cast checks if the basePtr can be safely cast to a Derived*. Since basePtr actually points to a Derived object, the cast succeeds, and we see "Derived class" printed.
Const Cast in C++ to Modify Constant Values
Const_cast is our tool when we need to change a constant value or remove the constness of a variable. It’s powerful but should be used carefully since it breaks the promise of const correctness.
Example: Modifying a Constant Variable Using Const Cast
#include using namespace std;
void updateValue(const int* ptr) { int* modifiablePtr = const_cast *modifiablePtr = 100; }
int main() { int num = 50; const int* numPtr = #
updateValue(numPtr);
cout << "Updated Value: " << num << endl; return 0; } |
Output:
Updated Value: 100 |
In this example, we use const_cast to modify the value of num even though it’s accessed through a const int* pointer.
Reinterpret Cast in C++ for Low-Level Data Reinterpretation
Reinterpret_cast is the most flexible, but also the riskiest.
It lets us convert any pointer type to any other pointer type, but it doesn’t check whether the cast is valid.
We should use it only when absolutely necessary, like when working with hardware or performing certain optimizations.
Example: Converting Pointer Types with Reinterpret Cast in C++
#include using namespace std;
int main() { int num = 97; char* charPtr = reinterpret_cast cout << "Character Value: " << *charPtr << endl; return 0; } |
Output:
Character Value: a |
Here, reinterpret_cast converts an int pointer to a char pointer, allowing us to interpret the memory as a different type. This can be useful in low-level programming but should be handled with care to avoid undefined behaviour.
Common Pitfalls and Best Practices in Type Conversion
Type conversion in C++ is very powerful but, at the same time, risky.
We have to be aware of the possible pitfalls and try to follow the best practices mentioned in the C++ tutorial so that our code will be safe and efficient.
Watch out for these common issues:
- Data loss: Conversion from some bigger data types into a smaller data type may result in losing data during the conversion. This can happen when converting a double to an int.
- Unpredicted Behavior: Usually, it can create unexpected results if not taken into account, especially when mixed with types.
- Undefined Behavior: Using reinterpret_cast or casting between incompatible types can lead to undefined behaviour.
Best practices to follow:
- Use Explicit Casts: Use explicit casts, like static_cast, dynamic_cast, etc., to indicate that the conversions are clear and intentional.
- Avoid Unnecessary Conversions: Conversions should be minimised as much as possible to reduce the likelihood of mistakes.
- Check Validity: When using dynamic_cast, always check if the cast was successful before using the result.
Comparison of Different Cast Operators in C++
Choosing the right cast operator is crucial. Here’s a quick comparison:
- Static Cast: Use when converting between related types, and you’re sure of the conversion.
- Dynamic Cast: Use in polymorphic scenarios where you need to ensure the conversion is safe at runtime.
- Const Cast: Use sparingly only when you need to modify a const value.
- Reinterpret Cast: Use with caution only when you need to perform low-level data manipulation.